Counting Characters
March 3, 2024 11:07 pm
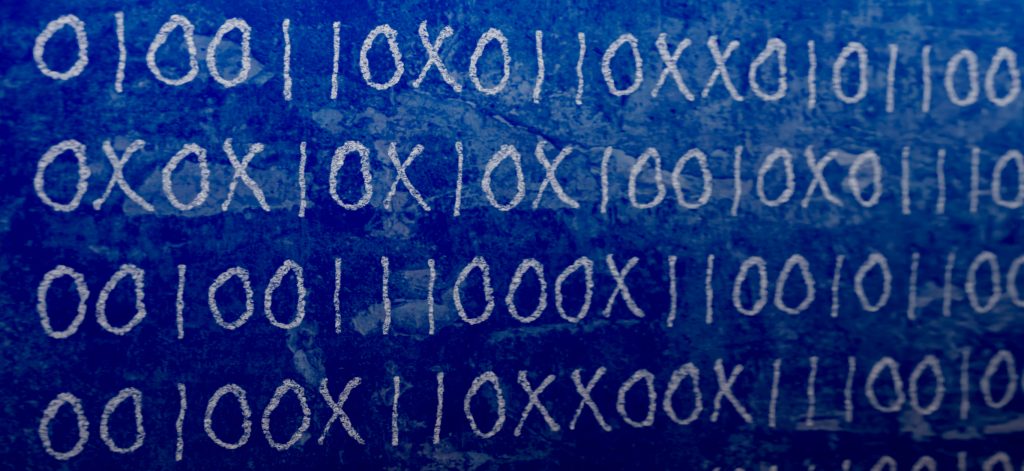
I was looking into Nanyan numerals again, and I found some interesting things. I was optimizing the algorithm I was using and running analysis, and one of the things I was thinking about was how many characters it takes to write a given numeral.
NOTE: For this post, I will be using X for the “2 x” character instead of Θ, and all numerals will be written left to right.
The upper-bound for any number of characters is easy to figure out: it has to be a power of two. Every power of two contains one O and then X’s so that the total number of characters is the power two is raised to. Therefore, since 8 is 2 ^ 3, the Nanyan numeral for eight is OXX. This is the last numeral containing three characters, just as 16 is the last with four and 32 is the last with five.
So that’s easy powers of 2, but there is an easy algorithm for determining the number of characters for any number. It turns out that it’s practically the same as generating the numeral. Here it is:
Start with the number, say 30, and find the lowest factor for that number, in this case 2. Then divide the number by that factor and subtract: 30 – 15 = 15. (For prime numbers, subtract 1.) Now keep going until you reach 1. Like this:
30 – 15 = 15
15 – 5 = 10
10 – 5 = 5
5 – 1 = 4
4 – 2 = 2
2 – 1 = 1
Count the steps, and you’ll find that 30 has 6 characters: OO|OX|
Actually, this is another algorithm for finding the characters necessary for the construction of the numeral, but out of order:
30 – 15 = 15 -> 2 -> O
15 – 5 = 10 -> 3 -> |
10 – 5 = 5 -> 2 -> O
5 – 1 = 4 -> 5 -> |
4 – 2 = 2 -> 2 -> O
2 – 1 = 1 -> 2 -> X
The trick is at each step, the characters are in reverse, though the order of the primes is correct. It gets more complex for larger primes that create more recursions. (A program for this algorithm is forthcoming.)
The biggest resource that helped me realize these things was The On-Line Encyclopedia of Integer Sequences (OEIS). (NOTE: I have no affiliation with the OEIS). After running some scripts I wrote analyzing character length for various numerals, I ran those numbers through the OEIS just to see if there were any corresponding sequences, and these are what I found:
- Number of Characters Used to Write a Numeral (the only wrong value is for 1)
- Lowest Number of a Given Character Length (again, 1 is wrong)
- Number of Nanyan Numerals of a Given Length (again, 1 must be accounted for because 1 can be written with one character)
Comments